✔︎ Last updated on November 11th, 2024
Lightening or darkening a color is a common use case in UI design. You may want to darken the background color when a user hovers over a button, or you may use a slightly lighter shade of the text color for the background of an alert.
In this article, we will start with the usual approach and then we will look at how we can reduce reliance on external tools and use the native color formats to achieve the desired effect.
Let’s go.
The usual approach to lightening or darkening colors in CSS
One of the traditional approaches to darkening or lightening colors is to use CSS preprocessors like SASS or SCSS. For example, there are functions called lighten
and darken
in SCSS which take two arguments: color and percentage (by which to lighten or darken it).
.danger-alert {
color: red;
// light background color of same hue
background-color: lighten(red, 40%);
}
button:hover {
// darken the background color on hover
background-color: darken(#eee, 10%);
}
This approach obviously works and is great if you already use these preprocessors.
Another approach is to manually find the darker variant of the same color using an online tool such as this one and then hardcode it in your CSS.
This is how these tools work: you feed in the hex value of a color in the input box below and it will generate 10 versions of this color, each one darker than the previous one by 5%.
See the Pen Untitled by Gauri Shanker (@Gsbansal) on CodePen.
Also read: 17+ Awesome Excalidraw Tips for Insane Productivity
What if I told you there was a much better and more intuitive way to darken and lighten colors by a fixed percentage? Plus, you can use this method in CSS natively without relying on any external preprocessor.
Changing the lightness and brightness of colors in CSS natively
A better approach is using the HSL color format over other formats. HSL color format represents colors in a way that is more intuitive for humans.
HSL format takes three parameters (four if you count the opacity value):
H (Hue): Which color hue do you want (ranges between 0 and 359 degrees on a color wheel)
S (Saturation): How much of hue in that color (between 0% and 100%)
L (Lightness): How bright or dark do you want the color to be (0% means completely dark or black, 50%: pure color, 100%: pure white)
Alpha (opacity): How transparent you want the color to be. (Optional)
I hope you’re beginning to grasp how effortlessly you can adjust the intensity of colors. Once you’ve chosen a specific hue and saturation, creating lighter or darker versions of the color is a breeze. It’s as simple as tweaking the third parameter—Lightness.
Also read: How to Change the Default Fonts in Excalidraw
Need a brighter background? Just dial up the lightness. Looking for a darker shade? Lower the lightness. It’s that straightforward!
👉 LIVE DEMO
In the demo below, try adjusting the ‘Lightness’ slider and see how the div
below shows all the shades of the same color:
This feature of the HSL color format stands in stark contrast to the RGB format, where darker shades of the same color have entirely different values that can be unpredictable to the human eye.
For instance, observe how these five shades of red have clear and understandable values in the HSL format, while their values in the HEX format can be entirely unpredictable.
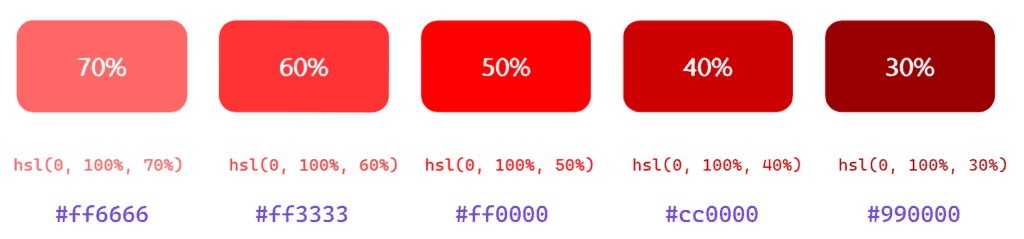
button {
color: hsl(230, 100%, 50%); /* text color */
background-color: hsl(230, 100%, 80%); /* lighter background */
}
Output:
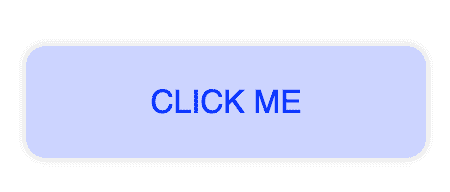
Now let’s see how you can accomplish some basic tasks with pure CSS using the HSL color format:
Darken color on hover in CSS
Suppose I have a light red div
in HTML:
<div class="red"></div>
.red {
height: 300px;
width: 300px;
background-color: hsl(0, 100%, 70%); /* light red */
}
Now, I want to darken its background color when I hover it with the cursor. I can simply dial down the third parameter (lightness) in the hsl
color format from 70% to 40%.
.red {
height: 300px;
width: 300px;
background-color: hsl(0, 100%, 70%);
}
/* darken it on hover */
.red:hover{
background-color: hsl(0, 100%, 30%);
}
For a smoother transition, add a transition duration of 0.5s
. This is what it looks like when run on Codepen —
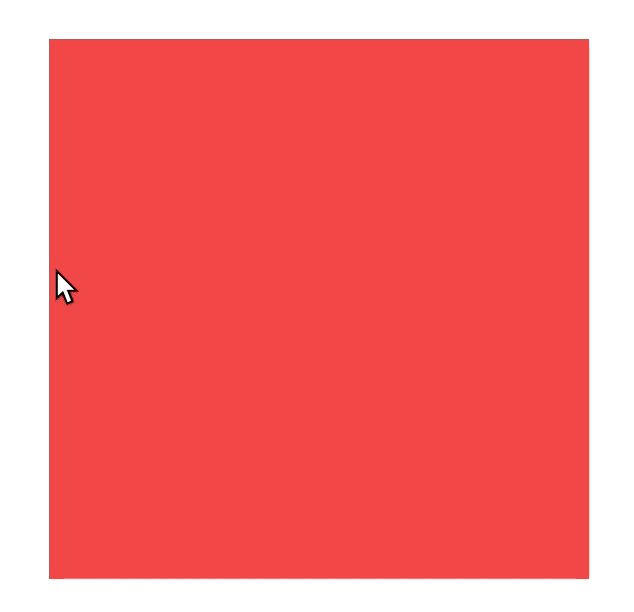
Lighten color in CSS
Similar to darkening a color in CSS, lightening it is also a breeze. You just have to ramp up the ‘lightness’ parameter in the hsl
color format.
/* from this */
div {
background-color: hsl(0, 100%, 30%);
}
/* to this */
div:hover {
background-color: hsl(0, 100%, 60%);
}
Another approach to lightening or darkening colors in CSS involves using the brightness filter in CSS. Here’s how the above examples can be modified to darken or lighten colors on hover:
/* from this */
div {
background-color: hsl(0, 100%, 60%);
}
/* to this */
div:hover {
filter: brightness(70%);
}
This line of code will clamp the brightness of the element to 70% of its maximum value when it is hovered.
Please remember that the brightness filter affects the entire element, not just the background-color. So, this means that everything within that element, including any children elements, will also be affected. This is particularly useful if you have a video or image that you want to adjust the brightness of, as the brightness filter will work perfectly for that.
Fading a color with HSL in CSS
Saturation, the second parameter in the HSL color format, is also a pretty handy tool in UI design.
You can ramp it up or dial it down which will change the vibrancy of a color in an element. And, when you tweak it alongside the Lightness parameter, setting color values becomes surprisingly intuitive.
For example, you can create a disabled button look by lowering the saturation and increasing the brightness.
<div class="container">
<button>Normal Button</button>
<button disabled>Disabled Button</button>
</div>
/* Basic styles not shown */
/* Tutorial styles 👇 */
button {
background: hsl(240, 100%, 60%);
}
/* lower the saturation, increase the lightness */
button:disabled {
background: hsl(240, 20%, 80%);
}
Output:
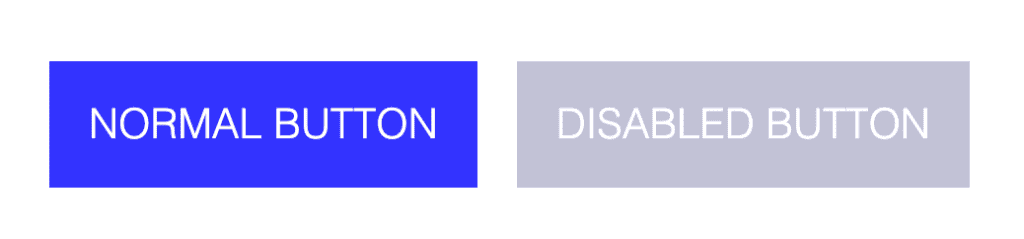
I hope you learned how you can lighten or darken colors in CSS without using any preprocessor or external tool.
If you found this article useful, please share it with your friends. If you have a better approach, please let me know by dropping a line below.
FAQs
How to Lighten or Darken a Color Using CSS?
Lightening or darkening a color in CSS involves adjusting the “lightness” parameter of the HSL color format. This parameter ranges from 0% (black) to 100% (white). By increasing the lightness value, you can make the color lighter and by decreasing it, you can darken a color.
Other approaches to darkening or lightening colors involves the use of built-in lighten
and darken
functions in CSS preprocessors such as SCSS, SCSS or LESS.
What are the benefits of using HSL over RGB for adjusting color brightness in CSS?
While both HSL and RGB work for modifying color brightness, HSL offers a more intuitive approach when adjusting lightness specifically. By focusing directly on the “lightness” parameter, you have more control over how much brighter or darker a color becomes, making it easier to maintain visual relationships between colors in your design.
How to darken a color on hover in CSS?
To darken a color on hover, first define a base color in the hsl
format in the element’s declarations. Then, on the property declarations with the :hover
pseudo class, declare the property named color
or background-color
and use the hsl
color parameter. In these declarations, tone down the value of the third parameter (lightness
).
Here’s a sample code you can take inspiration from:
How to darken the background color with CSS?
To darken the background color with pure CSS, you can apply HSL color adjustments and keeping the third parameter (lightness) to a lower value:
background-color: hsl(180, 50%, 30%);
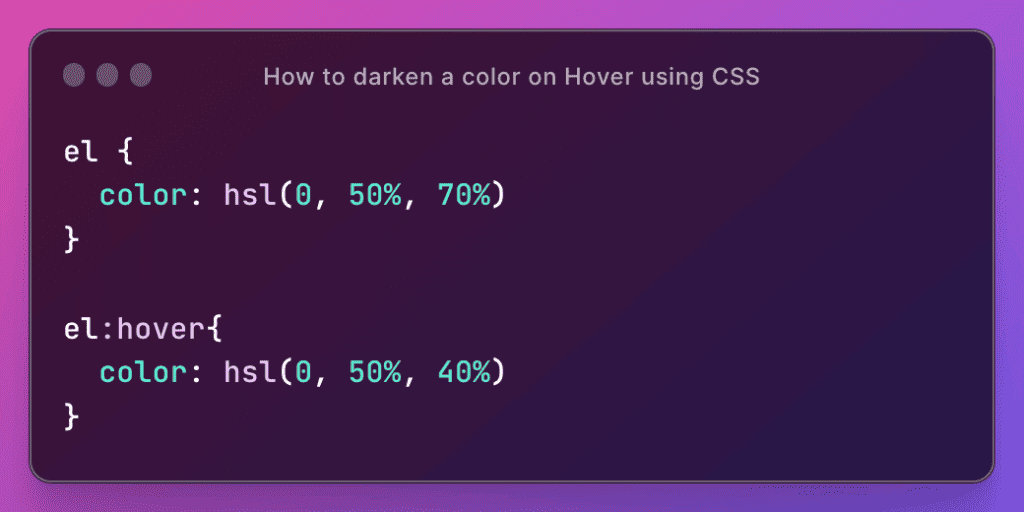
Thanks for reading. 🍻
To optically lighten or darken a color wouldn´t it be better to use okhsl() or even oklch()? certainly when contrasting colors in fore and background?